I am making a program that opens and reads a file.
This is my code:
import java.io.*;
public class FileRead{
public static void main(String[] args){
try{
File file = new File("hello.txt");
System.out.println(file.getCanonicalPath());
FileInputStream ft = new FileInputStream(file);
DataInputStream in = new DataInputStream(ft);
BufferedReader br = new BufferedReader(new InputStreamReader(in));
String strline;
while((strline = br.readLine()) != null){
System.out.println(strline);
}
in.close();
}catch(Exception e){
System.err.println("Error: " + e.getMessage());
}
}
}
but when I run, I get this error:
C:UsersUserDocumentsWorkspaceFileReadhello.txt
Error: hello.txt (The system cannot find the file specified)
my FileRead.java
and hello.txt
where in the same directory that can be found in:
C:UsersUserDocumentsWorkspaceFileRead
I’m wondering what I am doing wrong?
eddie
1,2523 gold badges15 silver badges20 bronze badges
asked Jul 19, 2012 at 2:18
2
Try to list all files’ names in the directory by calling:
File file = new File(".");
for(String fileNames : file.list()) System.out.println(fileNames);
and see if you will find your files in the list.
answered Jul 19, 2012 at 2:23
Eng.FouadEng.Fouad
115k70 gold badges312 silver badges416 bronze badges
3
I have copied your code and it runs fine.
I suspect you are simply having some problem in the actual file name of hello.txt, or you are running in a wrong directory. Consider verifying by the method suggested by @Eng.Fouad
answered Jul 19, 2012 at 2:30
Adrian ShumAdrian Shum
38.6k10 gold badges81 silver badges131 bronze badges
2
You need to give the absolute pathname to where the file exists.
File file = new File("C:\Users\User\Documents\Workspace\FileRead\hello.txt");
answered Jul 19, 2012 at 2:27
In your IDE right click on the file you want to read and choose «copy path»
then paste it into your code.
Note that windows hides the file extension so if you create a text file «myfile.txt» it might be actually saved as «myfile.txt.txt»
answered Jan 31, 2014 at 10:44
moshikmoshik
1,4241 gold badge10 silver badges6 bronze badges
Generally, just stating the name of file inside the File constructor means that the file is located in the same directory as the java file. However, when using IDEs like NetBeans and Eclipse i.e. not the case you have to save the file in the project folder directory. So I think checking that will solve your problem.
answered Jul 1, 2014 at 16:50
How are you running the program?
It’s not the java file that is being ran but rather the .class file that is created by compiling the java code. You will either need to specify the absolute path like user1420750 says or a relative path to your System.getProperty("user.dir")
directory. This should be the working directory or the directory you ran the java command from.
mtk
13.1k16 gold badges72 silver badges112 bronze badges
answered Jul 19, 2012 at 3:05
km1km1
2,3731 gold badge21 silver badges27 bronze badges
1
When you run a jar, your Main class itself becomes args[0] and your filename comes immediately after.
I had the same issue: I could locate my file when provided the absolute path from eclipse (because I was referring to the file as args[0]). Yet when I run the same from jar, it was trying to locate my main class — which is when I got the idea that I should be reading my file from args[1].
4444
3,54110 gold badges32 silver badges43 bronze badges
answered Jul 1, 2014 at 16:45
sivaramsivaram
311 silver badge5 bronze badges
First Create folder same as path which you Specified. after then create File
File dir = new File("C:\USER\Semple_file\");
File file = new File("C:\USER\Semple_file\abc.txt");
if(!file.exists())
{
dir.mkdir();
file.createNewFile();
System.out.println("File,Folder Created.);
}
answered Dec 20, 2017 at 6:37
BholaBhola
3921 silver badge15 bronze badges
Put the word.txt directly as a child of the project root folder and a peer of src
Project_Root
src
word.txt
Disclaimer: I’d like to explain why this works for this particular case and why it may not work for others.
Why it works:
When you use File
or any of the other FileXxx
variants, you are looking for a file on the file system relative to the «working directory». The working directory, can be described as this:
When you run from the command line
C:EclipseWorkspaceProjectRootbin > java com.mypackage.Hangman1
the working directory is C:EclipseWorkspaceProjectRootbin
. With your IDE (at least all the ones I’ve worked with), the working directory is the ProjectRoot
. So when the file is in the ProjectRoot
, then using just the file name as the relative path is valid, because it is at the root of the working directory.
Similarly, if this was your project structure ProjectRootsrcword.txt
, then the path "src/word.txt"
would be valid.
Why it May not Work
For one, the working directory could always change. For instance, running the code from the command line like in the example above, the working directory is the bin
. So in this case it will fail, as there is not binword.txt
Secondly, if you were to export this project into a jar, and the file was configured to be included in the jar, it would also fail, as the path will no longer be valid either.
That being said, you need to determine if the file is to be an embedded-resource (or just «resource» — terms which sometimes I’ll use interchangeably). If so, then you will want to build the file into the classpath, and access it via an URL. First thing you would need to do (in this particular) case is make sure that the file get built into the classpath. With the file in the project root, you must configure the build to include the file. But if you put the file in the src
or in some directory below, then the default build should put it into the class path.
You can access classpath resource in a number of ways. You can make use of the Class
class, which has getResourceXxx
method, from which you use to obtain classpath resources.
For example, if you changed your project structure to ProjectRootsrcresourcesword.txt
, you could use this:
InputStream is = Hangman1.class.getResourceAsStream("/resources/word.txt");
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
getResourceAsStream
returns an InputStream
, but obtains an URL under the hood. Alternatively, you could get an URL
if that’s what you need. getResource()
will return an URL
For Maven users, where the directory structure is like src/main/resources
, the contents of the resources
folder is put at the root of the classpath. So if you have a file in there, then you would only use getResourceAsStream("/thefile.txt")
In this tutorial, we will discuss how to solve the java.io.FileNotFoundException – FileNotFoundException
in Java. This exception is thrown during a failed attempt to open the file denoted by a specified pathname.
Also, this exception can be thrown when an application tries to open a file for writing, but the file is read-only, or the permissions of the file do not allow the file to be read by any application.
You can also check this tutorial in the following video:
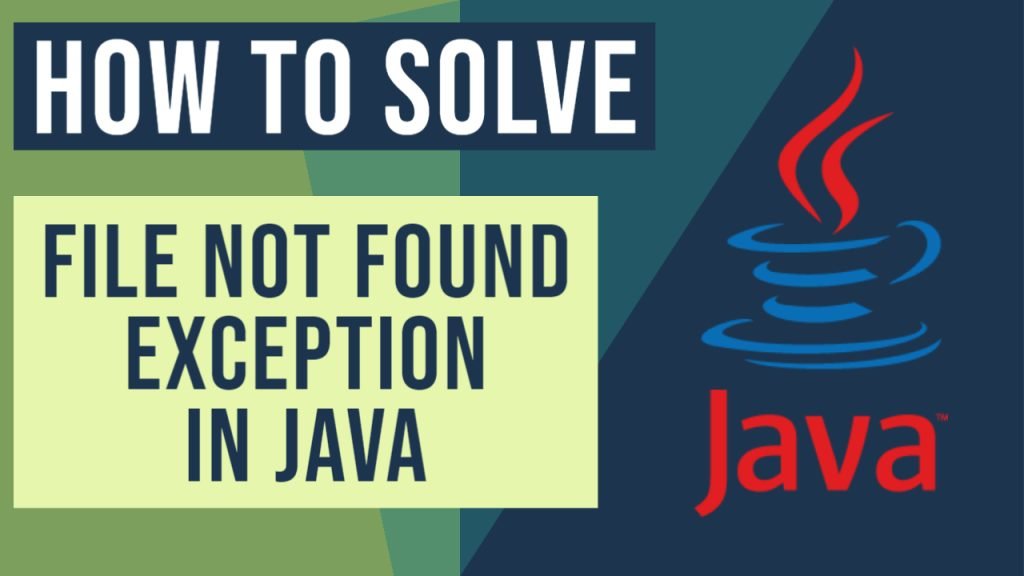
This exception extends the IOException
class, which is the general class of exceptions produced by failed or interrupted I/O operations. Also, it implements the Serializable
interface and finally, the FileNotFoundException
exists since the first version of Java (1.0).
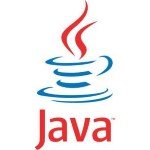
The following constructors throw a FileNotFoundException
when the specified filename does not exist: FileInputStream
, FileOutputStream
, and RandomAccessFile
. These classes aim to obtain input bytes from a file in a file system, while the former class supports both reading and writing to a random access file.
The following snippet reads all the lines of a file, but if the file does not exist, a java.io.FileNotFoundException is thrown.
FileNotFoundExceptionExample.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
|
In case the file is missing, the following output is produced:
An IOException was caught! java.io.FileNotFoundException: input.txt (No such file or directory) at java.io.FileInputStream.open(Native Method) at java.io.FileInputStream.(FileInputStream.java:146) at java.io.FileReader.(FileReader.java:72) at main.java.FileNotFoundExceptionExample.main(FileNotFoundExceptionExample.java:16) Exception in thread "main" java.lang.NullPointerException at main.java.FileNotFoundExceptionExample.main(FileNotFoundExceptionExample.java:30)
The following snippet tries to append a string at the end of a file. If the file does not exist, the application creates it. However, if the file cannot be created, is a directory, or the file already exists but its permissions are sufficient for changing its content, a FileNotFoundException
is thrown.
FileNotFoundExceptionExample_v2.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
|
If the file exists and is a directory, the following exception is thrown:
An IOException was caught! java.io.FileNotFoundException: input.txt (Is a directory) at java.io.FileOutputStream.open(Native Method) at java.io.FileOutputStream.(FileOutputStream.java:221) at java.io.FileWriter.(FileWriter.java:107) at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:16) Exception in thread "main" java.lang.NullPointerException at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:28)
If the file exists, but it doesn’t have the appropriate permissions for writing, the following exception is thrown:
An IOException was caught! java.io.FileNotFoundException: input.txt (Permission denied) at java.io.FileOutputStream.open(Native Method) at java.io.FileOutputStream.(FileOutputStream.java:221) at java.io.FileWriter.(FileWriter.java:107) at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:16) Exception in thread "main" java.lang.NullPointerException at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:28)
Finally, the aforementioned exception can occur when the requested file exists, but it is already opened by another application.
2. How to deal with the java.io.filenotfoundexception
- If the message of the exception claims that there is no such file or directory, then you must verify that the specified is correct and actually points to a file or directory that exists in your system.
- If the message of the exception claims that permission is denied then, you must first check if the permissions of the file are correct and second, if the file is currently being used by another application.
- If the message of the exception claims that the specified file is a directory, then you must either alter the name of the file or delete the existing directory (if the directory is not being used by an application).
Important: For those developers that use an IDE to implement their Java applications, the relative path for every file must be specified starting from the level where the src
directory of the project resides.
3. Download the Eclipse Project
This was a tutorial about the FileNotFoundException
in Java.
Last updated on Oct. 12th, 2021
Sotirios-Efstathios (Stathis) Maneas is a PhD student at the Department of Computer Science at the University of Toronto. His main interests include distributed systems, storage systems, file systems, and operating systems.
java.io.FileNotFoundException which is a common exception which occurs while we try to access a file. FileNotFoundExcetion is thrown by constructors RandomAccessFile, FileInputStream, and FileOutputStream. FileNotFoundException occurs at runtime so it is a checked exception, we can handle this exception by java code, and we have to take care of the code so that this exception doesn’t occur.
Declaration :
public class FileNotFoundException extends IOException implements ObjectInput, ObjectStreamConstants
Constructors :
- FileNotFoundException() : It gives FileNotFoundException with null message.
- FileNotFoundException(String s) : It gives FileNotFoundException with detail message.
It doesn’t have any methods. Now let’s understand the hierarchy of this class i.e FileNotFoundException extends IOException which further extends the Exception class which extends the Throwable class and further the Object class.
Hierarchy Diagram:
Why this Exception occurs?
There are mainly 2 scenarios when FileNotFoundException occurs. Now let’s see them with examples provided:
- If the given file is not available in the given location then this error will occur.
- If the given file is inaccessible, for example, if it is read-only then you can read the file but not modify the file, if we try to modify it, an error will occur or if the file that you are trying to access for the read/write operation is opened by another program then this error will occur.
Scenario 1:
If the given file is not available in the given location then this error will occur.
Example:
Java
import
java.io.*;
public
class
Example1
{
public
static
void
main(String[] args)
{
FileReader reader =
new
FileReader(
"file.txt"
);
BufferedReader br =
new
BufferedReader(reader);
String data =
null
;
while
((data = br.readLine()) !=
null
)
{
System.out.println(data);
}
br.close();
}
}
Output
prog.java:14: error: unreported exception FileNotFoundException; must be caught or declared to be thrown FileReader reader = new FileReader("file.txt"); ^ prog.java:25: error: unreported exception IOException; must be caught or declared to be thrown while ((data = br.readLine()) != null) ^ prog.java:31: error: unreported exception IOException; must be caught or declared to be thrown br.close(); ^ 3 errors
Scenario 2:
If the given file is inaccessible, for example, if it is read-only then you can read the file but not modify the file if we try to modify it, an error will occur or if the file that you are trying to access for the read/write operation is opened by another program then this error will occur.
Example:
Java
import
java.io.*;
import
java.util.*;
class
Example2 {
public
static
void
main(String[] args) {
try
{
File f=
new
File(
"file.txt"
);
PrintWriter p1=
new
PrintWriter(
new
FileWriter(f),
true
);
p1.println(
"Hello world"
);
p1.close();
f.setReadOnly();
PrintWriter p2=
new
PrintWriter(
new
FileWriter(
"file.txt"
),
true
);
p2.println(
"Hello World"
);
}
catch
(Exception ex) {
ex.printStackTrace();
}
}
}
Output
java.security.AccessControlException: access denied ("java.io.FilePermission" "file.txt" "write") at java.base/java.security.AccessControlContext.checkPermission(AccessControlContext.java:472) at java.base/java.security.AccessController.checkPermission(AccessController.java:897) at java.base/java.lang.SecurityManager.checkPermission(SecurityManager.java:322) at java.base/java.lang.SecurityManager.checkWrite(SecurityManager.java:752) at java.base/java.io.FileOutputStream.<init>(FileOutputStream.java:225) at java.base/java.io.FileOutputStream.<init>(FileOutputStream.java:187) at java.base/java.io.FileWriter.<init>(FileWriter.java:96) at Example2.main(File.java:19)
Handling Exception:
Firstly we have to use the try-catch block if we know whether the error will occur. Inside try block all the lines should be there if there are chances of errors. There are other remedies to handle the exception:
- If the message of the exception tells that there is no such file or directory, then you re-verify whether you mentioned the wrong file name in the program or file exists in that directory or not.
- If the message of the exception tells us that access is denied then we have to check the permissions of the file (read, write, both read and write) and also check whether that file is in use by another program.
- If the message of the exception tells us that the specified file is a directory then you must either delete the existing directory(if the directory not in use) or change the name of the file.
Last Updated :
16 Nov, 2021
Like Article
Save Article
У меня есть имя файла с именем «first.java», сохраненное на моем рабочем столе в блокноте ++. Когда я запускаю команду cmd «javac first.java», она дает мне эту ошибку.
javac: файл не найден: first.java Использование: javac
Я знаю, что вам нужно перейти на C:Programfilesjavajdk.
и в моем C:Program FilesJavaу меня есть папки thesE
«jdk1.8.0»
«Jre6»
«Jre8»
В C:Program Files (x86)Java
У меня есть эта папка
«Jre6»
Настройки окружающей среды выглядят следующим образом
CLASSPATH
C:Program FilesJavajre8bin
Имя переменной: LEJOS_NXT_JAVA_HOME
Значение переменной: C:Program Files (x86)Javajdk1.6.0_21bin
PATH
Имя переменной: PATH
Значение переменной: C:Program FilesJavajdk1.8.0bin
Скажите, пожалуйста, где я ошибаюсь. Я прочитал несколько сообщений в Интернете, и я не могу понять это.
14 дек. 2013, в 22:02
Поделиться
Источник
11 ответов
Как указано nAvEeD kHaN, вам нужно указать свой путь.
Но для того, чтобы ваш JDK мог воспользоваться такими инструментами, как Maven в будущем, я бы назначил путь JAVA_HOME
к вашей папке JDK, а затем просто добавлю каталог %JAVA_HOME%bin
в ваш PATH
.
JAVA_HOME = "C:Program FilesJavajdk1.8.0"
PATH = %PATH%%JAVA_HOME%bin
В Windows:
- right-click «Мой компьютер» и выберите «свойства» из контекстного меню.
- Выберите «Расширенные системные настройки» слева.
- В всплывающем окне «Свойства системы» на вкладке «Дополнительно» выберите «Переменные среды»
- В всплывающем окне «Переменные среды» в разделе «Системные переменные» нажмите «Создать»
- Введите « JAVA_HOME» в поле «Имя переменной»
- Вставьте путь к папке JDK в поле «Значение переменной».
- Нажмите OK
- В этом же разделе выберите «Путь» и измените текст, где он говорит:
blah;blah;blah;
C:Program FilesJavajdk1.8.0bin; blah,blah;blah
к:
blah;blah;blah;
% JAVA_HOME%бин; blah,blah;blah
Mr. Polywhirl
14 дек. 2013, в 20:37
Поделиться
SET путь JRE также
jre — не что иное, как ответственность за выполнение программы
PATH Значение переменной:
C:Program FilesJavajdk1.8.0bin;C:Program FilesJavajrebin;.;
KhAn SaHaB
14 дек. 2013, в 21:55
Поделиться
У меня была такая же проблема, и это связано с моим именем файла. Если вы установили местоположение файла с помощью CD в CMD, а затем введите DIR, он перечислит файлы в этом каталоге. Убедитесь, что имя файла отображается и проверьте правильность написания и имени файла.
Это должен быть .java, но мой был .java.txt. В инструкциях на веб-сайте Java tutorials указывается, что вы должны выбрать «Сохранить как текстовые документы типа», но для меня это всегда добавляет .txt на конец имени файла. Если я изменил его на «Сохранить как все документы», он правильно сохранил имя файла.
Stephen Dryden
05 сен. 2015, в 18:28
Поделиться
Похоже, у вас есть правильный путь. Но каков ваш рабочий каталог?
при запуске командной строки:
javac -version
это должно показать вашу версию java. если это не так, вы не правильно установили java в пути.
перейдите к C:
cd
Тогда:
javac -sourcepath usersAccNameDesktop -d usersAccNameDesktop first.java
-sourcepath — это полный путь к вашему .java файлу, -d — это путь/каталог, в котором вы хотите, чтобы ваши файлы .class были, а затем, наконец, файл, который вы хотите скомпилировать (first.java).
Ramson Mwangi
29 янв. 2015, в 13:26
Поделиться
Для Windows javac
должна быть команда, которую вы можете запускать из любого места. Если это не так (что странно и само по себе), вам нужно запустить javac
с того места, где он находится, но перейти к точному местоположению вашего файла Java-класса, чтобы его скомпилировать успешно.
По умолчанию javac
будет компилировать имя файла относительно текущего пути, и если он не сможет найти файл, он не будет его компилировать.
Обратите внимание: вы действительно сможете использовать jdk1.8.0 для компиляции, поскольку это будет единственный набор библиотек, содержащий javac
, содержащийся в нем. Помните: Java Runtime Environment запускает классы Java; Java Development Kit компилирует их.
Makoto
14 дек. 2013, в 21:36
Поделиться
Процедура выполнения файла Java:
После сохранения файла MyFirstJavaProgram2.java
Введите весь путь «Javac», за которым следует java файл
Для выполнения вывода
Путь с последующим комментарием < -cp > за которым следует
Ниже приведен пример выполнения
C:Program FilesJavajdk1.8.0_101bin > javac C:SampleMyFirstJavaProgram2.java
C:Program FilesJavajdk1.8.0_101bin > java -cp C:Sample MyFirstJavaProgram2
Hello World
Beginner_PCR
16 фев. 2017, в 22:43
Поделиться
У меня была такая же проблема. Происхождение проблемы состояло в создании текстового файла в «Блокноте» и переименовании его в виде java файла. Это означало, что файл был сохранен как файл WorCount.java.txt.
Чтобы решить эту проблему, мне удалось сохранить файл в виде java файла в среде IDE или в Nodepad ++.
shareToProgress
15 окт. 2016, в 13:47
Поделиться
Итак, у меня была такая же проблема, потому что я не был в правильном каталоге, где находился мой файл. Поэтому, когда я побежал javac Example.java
(для меня), он сказал, что не может найти его. Но мне нужно было пойти туда, где был найден мой файл Example.java
. Поэтому я использовал команду cd
и сразу после этого разместил файл. Это сработало для меня. Скажи мне, если это поможет! Благодарю!
Jai Bansal
17 авг. 2015, в 17:24
Поделиться
Вот как я выполнил программу без настройки переменной среды.
Процедура выполнения файла Java:
После сохранения файла MyFirstJavaProgram.java
Введите весь путь «Javac», за которым следует java файл
Для выполнения вывода
Путь с последующим комментарием < -cp > за которым следует
Ниже приведен пример выполнения
C:Program FilesJavajdk1.8.0_101bin > javac C:SampleMyFirstJavaProgram2.java
C:Program FilesJavajdk1.8.0_101bin > java -cp C:Sample MyFirstJavaProgram2
Hello World
Beginner_PCR
16 фев. 2017, в 22:26
Поделиться
Сначала укажите путь к C:/Program Files/Java/jdk1.8.0/bin в переменных среды.
Скажем, ваш файл сохраняется на диске C:
Теперь откройте командную строку и перейдите в каталог с помощью c:
javac Filename.java
java Имя файла
Убедитесь, что вы сохранили имя файла как .java.
Если он сохранен в виде текстового файла, тогда файл не найден ошибкой, так как он не может использовать текстовый файл.
Android
23 окт. 2016, в 13:59
Поделиться
Если это проблема, перейдите туда, где присутствует javac, а затем введите
- блокнот Yourfilename.java
- он попросит создать файл
- скажите «да» и скопируйте код из предыдущего исходного файла в этот файл.
- теперь выполните cmd —— > javac Yourfilename.java
Это будет работать точно.
vinaykotagi
26 нояб. 2014, в 10:31
Поделиться
Ещё вопросы
- 1Получить дамп потока в браузере JS?
- 0Как показать Gmail вставленные изображения на моей веб-странице
- 1Как унаследовать всю функциональность родительского класса?
- 0Как реализовать одно и то же действие с переходом и без перехода?
- 1React Native: событие onContentSizeChange для элемента TextInput не работает на Android
- 1Эффективная фильтрация больших наборов многоключевых объектов
- 1LibSVM и Weka API не работали вместе
- 1Утверждение и документация в классе для методов, которые ожидаются в производных классах
- 0пытаясь заставить PHP разместить строку в файл
- 0Scanf и строки в C ++
- 1сохранить несколько каталогов изображений в одном файле после предварительной обработки
- 0Как найти количество тегов Div в HTML-файл в IOS?
- 1Повторение вызова метода
- 0Путаница с машинно-зависимыми значениями указателей
- 0Проверьте размеры файла изображения при вводе на стороне клиента AngularJS
- 0AngularJS: поделиться общей фабрикой для создания нового объекта
- 1Выходной файл не содержит все строки, которые я скопировал из источника
- 1Панды поворачивают один столбец, используя то же значение столбца, что и заголовки столбцов
- 0Отсутствуют заголовочные файлы для Tidy HTML для C
- 1Заголовок fetch () отображается как application / x-www-form-urlencoded вместо application / json
- 0GLUT взаимодействие с мышью
- 1Невозможно отправить настраиваемое поле, которое расширяет представление данных
- 0Шаблонная директива AngularJS с Java Play
- 0Оберните кучу вариантов внутри стола в Symfony / Twig
- 1NotificationCompat.Builder () не принимает идентификатор канала в качестве аргумента
- 0JavaScript, изменение изображения с анимацией
- 1Заполнение объекта при инициализации с использованием его конструктора и объекта, возвращенного из сериализованного потока
- 1Нужен ли семафор, когда один процесс пишет, а другой читает?
- 0Передача массива в качестве аргумента в параметризованный конструктор
- 0Выполнить код, когда отображается частичное представление
- 0мы можем использовать скомпилированный код hiphop на производственном сервере без исходного кода?
- 1Плавно отодвинуть твердое тело
- 0Изменить цвет фона из ввода
- 0PHP Невозможно просмотреть изображение при загрузке с клиента на сервер
- 0Функция JQuery Click () выполняется только один раз
- 1Ошибка распознавания токена в: ‘#i’ при разборе грамматики c
- 0Блокировка / замораживание таблицы в ListView
- 0найти элемент по названию в сортируемом — jquery
- 1ListView Windows 8, можем ли мы изменить поведение Swipe влево / вправо на Swipe Up / Down
- 1Как использовать + = (Добавить И) с пониманием списка
- 1Есть ли способ применить метод finalize ()?
- 0Список в C # контроллер от угловой
- 1Элемент управления WinForms WebBrowser игнорирует реферер
- 0синтаксическая ошибка в моих SQL-запросах
- 1колба — сессия юнит-тестирования с носом
- 1Задача «ConvertResourcesCases» неожиданно завершилась неудачей. Xamarin Android build
- 0Не удается заставить этот модульный тест (на помощнике) работать без исключений — AngularJS, Karma, Jasmine
- 0DIV перестает обновляться при создании новой записи в базе данных
- 0как разбирать в каком то улье?
- 0AngularJS — Лучший способ повторить создание cols